A Deep Dive into NgRx Effects: Managing Side Effects in Angular Applications
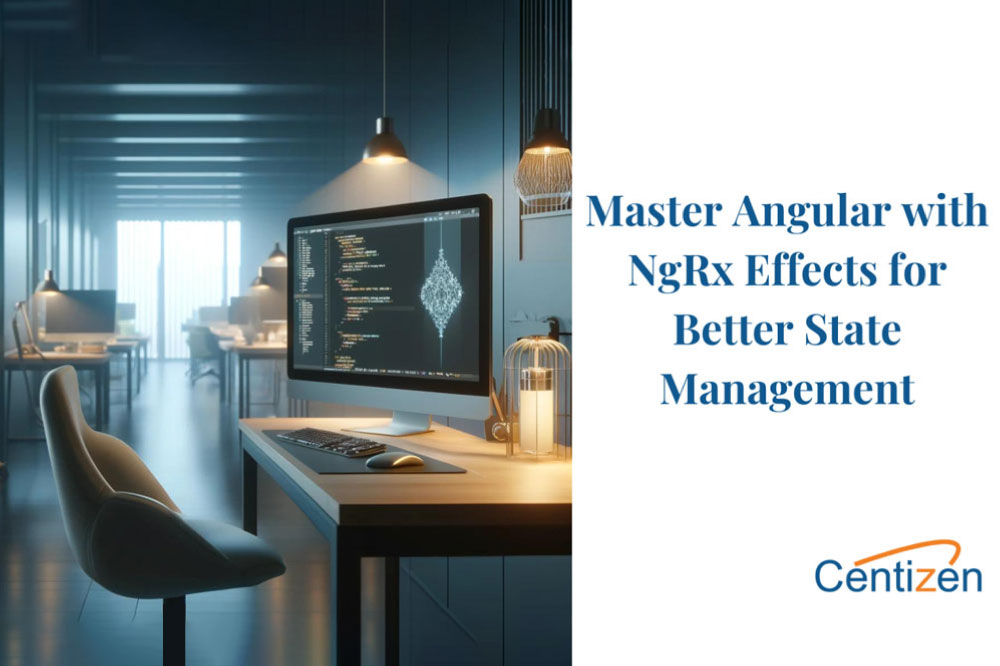
When developing complex applications with Angular, managing state and handling side effects efficiently can significantly impact your app’s responsiveness and user experience. NgRx, a framework inspired by Redux, provides robust solutions for state management in Angular applications. One of its powerful features is NgRx Effects, which handles side effects like data fetching, impure operations, and more. This blog post offers an in-depth exploration of NgRx Effects, demonstrating how to enhance your Angular applications by efficiently managing side effects.
Understanding NgRx effects
NgRx Effects is a library designed to handle side effects outside your Angular components and services. It listens for actions dispatched from your store, performs side effects, and returns new actions either immediately or asynchronously. This separation ensures your components and state management are clean, predictable, and easy to maintain.
Key concepts:
- Effects: Model side-effect logic as sources of actions.
- Actions: Plain JavaScript objects that communicate what change should happen.
- Reducers: Handle changes within your state based on the actions received.
- Store: The single truth source, maintaining the state of your application.
Setting up NgRx effects
To start using NgRx Effects in your Angular project, first install the necessary packages:
npm install @ngrx/store @ngrx/effects @ngrx/entity @ngrx/store-devtools
Next, set up the basic structure in your AppModule:
import { EffectsModule } from '@ngrx/effects';
import { StoreModule } from '@ngrx/store';
import { YourEffect } from './store/effects/your.effect';
@NgModule({
declarations: [...],
imports: [
StoreModule.forRoot(reducers),
EffectsModule.forRoot([YourEffect])
],
bootstrap: [AppComponent]
})
export class AppModule {}
Implementing a basic effect
Let’s create a simple effect that handles a data-loading operation when a specific action is dispatched:
import { Injectable } from '@angular/core';
import { Actions, ofType, createEffect } from '@ngrx/effects';
import { EMPTY } from 'rxjs';
import { map, mergeMap, catchError } from 'rxjs/operators';
import { DataService } from '../data.service';
import * as DataActions from './data.actions';
@Injectable()
export class DataEffects {
loadData$ = createEffect(() => this.actions$.pipe(
ofType(DataActions.loadRequest),
mergeMap(() => this.dataService.getAll()
.pipe(
map(data => DataActions.loadSuccess({ data })),
catchError(() => EMPTY)
))
)
);
constructor(
private actions$: Actions,
private dataService: DataService
) {}
}
In this example, loadData$ listens for loadRequest actions, calls a service to retrieve data, and dispatches a loadSuccess action with the data or an error action if the operation fails.
Advanced use cases
NgRx Effects can handle more complex scenarios:
- Debouncing: Prevent unnecessary API calls by debouncing actions.
- Listening for multiple actions: Handle multiple types of actions within a single effect.
- Long-running tasks: Manage tasks like web sockets or long-polling operations.
Best practices
To maximize the benefits of NgRx Effects, consider these best practices:
- Encapsulate side effects: Keep all side-effect logic within effects.
- Single responsibility: Each effect should handle a single type of action.
- Testing: Write unit tests for your effects to ensure they handle expected actions and produce expected outcomes.
Conclusion
NgRx Effects are a powerful tool for managing side effects in Angular applications. By separating side-effect handling from components and centralizing it in effects, you can create more maintainable and robust applications. As you become more familiar with NgRx Effects, you’ll be able to tackle more complex state management challenges, making your Angular applications cleaner and more efficient.
Explore Centizen Inc’s comprehensive staffing solutions, custom software development and innovative software offerings, including ZenBasket and Zenyo, to elevate your business operations and growth.
Centizen
A Leading IT Staffing, Custom Software and SaaS Product Development company founded in 2003. We offer a wide range of scalable, innovative IT Staffing and Software Development Solutions.
Contact Us
USA: +1 (971) 420-1700
Canada: +1 (971) 420-1700
India: +91 63807-80156
Email: contact@centizen.com
Our Services
Products
Contact Us
USA: +1 (971) 420-1700
Canada: +1 (971) 420-1700
India: +91 63807-80156
Email: contact@centizen.com